This tool came out of some frustration with the Blender Hotkey layouts. Blender has amazing functionality, but my fingers aren't flexible enough to keep up with the hotkeys, and I was really missing some more context to the right click menus available. I wanted a filtered, simple toolkit that was relevant to what I needed at that moment.
The main goal was to avoid interrupting the flow of modelling so I could focus on the problem at hand rather than either look up the functionality or spend the next few seconds scanning a right click menu for
that item.
I decided to take a stab at making a hotkeyed pie Menu. Where Blender suffers in the UI/UX department, it more than shines in how well it exposes it's UI functionality to Python (although selection and mesh manipulation is still a bit of a dark art compared to Maya's MEL and PyMel).
I also wanted to keep it pretty concise- the core idea behind the Pie menu was to keep it fast, and keep the cognitive load low. I think I may have ended up a little bit on the too many options side, but it's still usable without getting lost.
Here are some of the items I did a bit of extra legwork to get in:
Partial Edge Loop Selection:
What I wanted was a version of edge loop selection that would stop at UV seams or where the mesh poles. The UV seams were the biggest driver of this to make marking seams easier, but it's a handy modelling tool too.
I started with an example I looked up on
Stack Exchange about how the edge loop lookup code someone had written actually works. Essentially it relies on walking around a faces edges using linked loops, but has a bunch of caveats- the code as written works as intended on a cube or something where the loop actually, you know, loops, but when applied to something with borders the results are a bit weird...
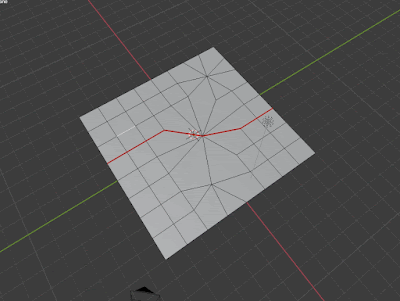 |
Nailed it. |
The algorithm has two termination conditions- either return back to the loop we started with and break, or reach the maximum iterations allowed and break.
To get to my intended result I added a couple of additional boundary termination clauses-
- if the radial loops to either side of the current loop are seams or borders, break.
- If one of the current loop's vertices aren't associated with exactly four faces, break.
This worked well, but to get the final result I was after, I also had to reverse my lookup direction if I hit one of the boundary clauses. This would give me partial and complete loops on either side of my connected edges.
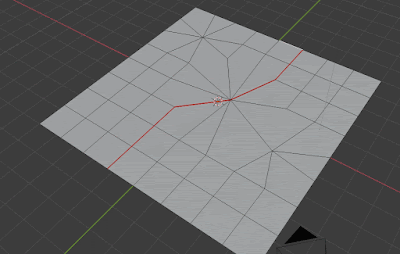 |
Partial edge loops are useful. |
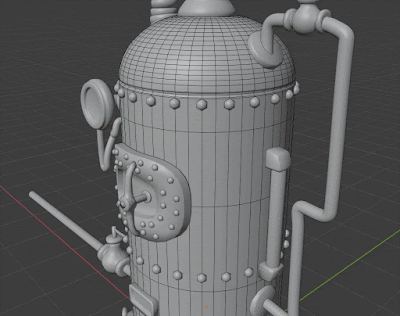 |
Partial edge loop selection in action. |
Handy tool! If you want to see more check the tool out
here.
-Pete